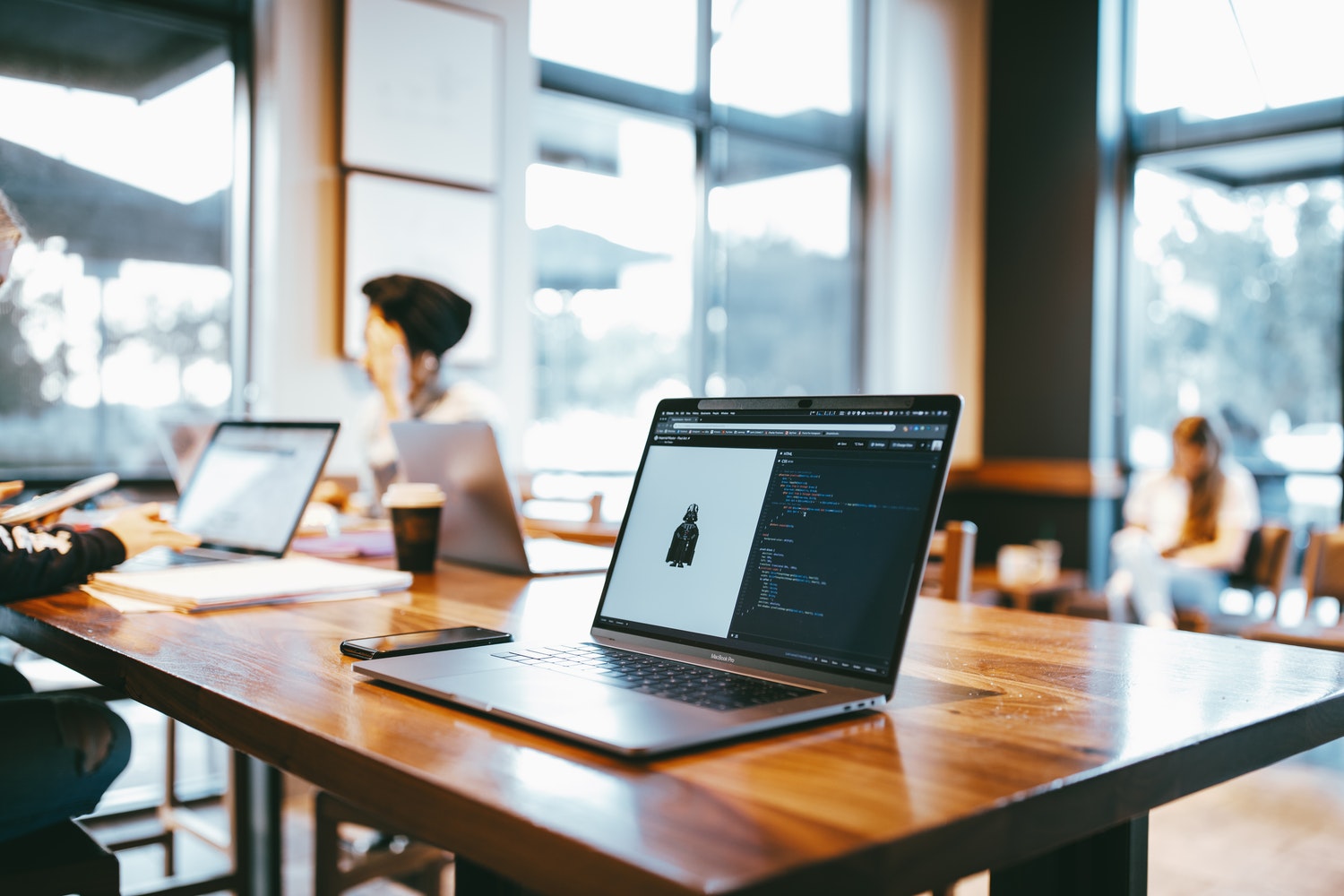
1 本节目标
- 代码规范
- 业务代码组织
- 首页代码编写
视频
代码
https://github.com/ducafecat/flutter_learn_news/releases/tag/v1.0.6
2 代码规范
2.1 官方代码规范
https://dart.dev/guides/language/effective-dart
2.3 chrome 插件 <彩云小译 - 网页翻译插件>
https://chrome.google.com/webstore/detail/lingocloud-web-translatio/jmpepeebcbihafjjadogphmbgiffiajh
2.4 阿里项目规范
3 业务界面代码组织
3.1 redux、fish-redux
- redux 架构
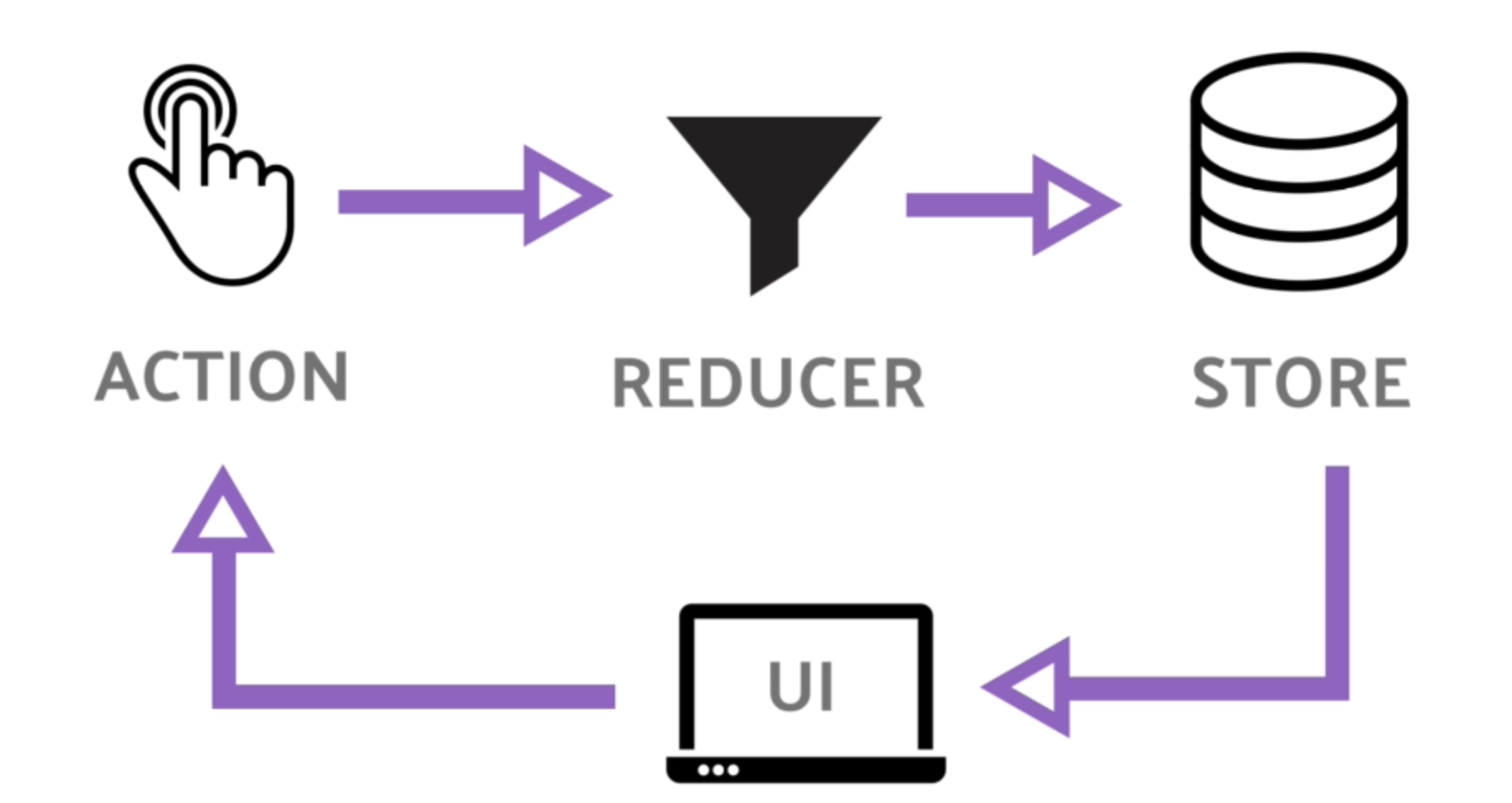
- fish-redux 架构
进一步的细分,进行规范
https://github.com/alibaba/fish-redux/tree/master/doc
https://medium.com/@dave790602/flutter-architecture-fish-redux-9b753912224a
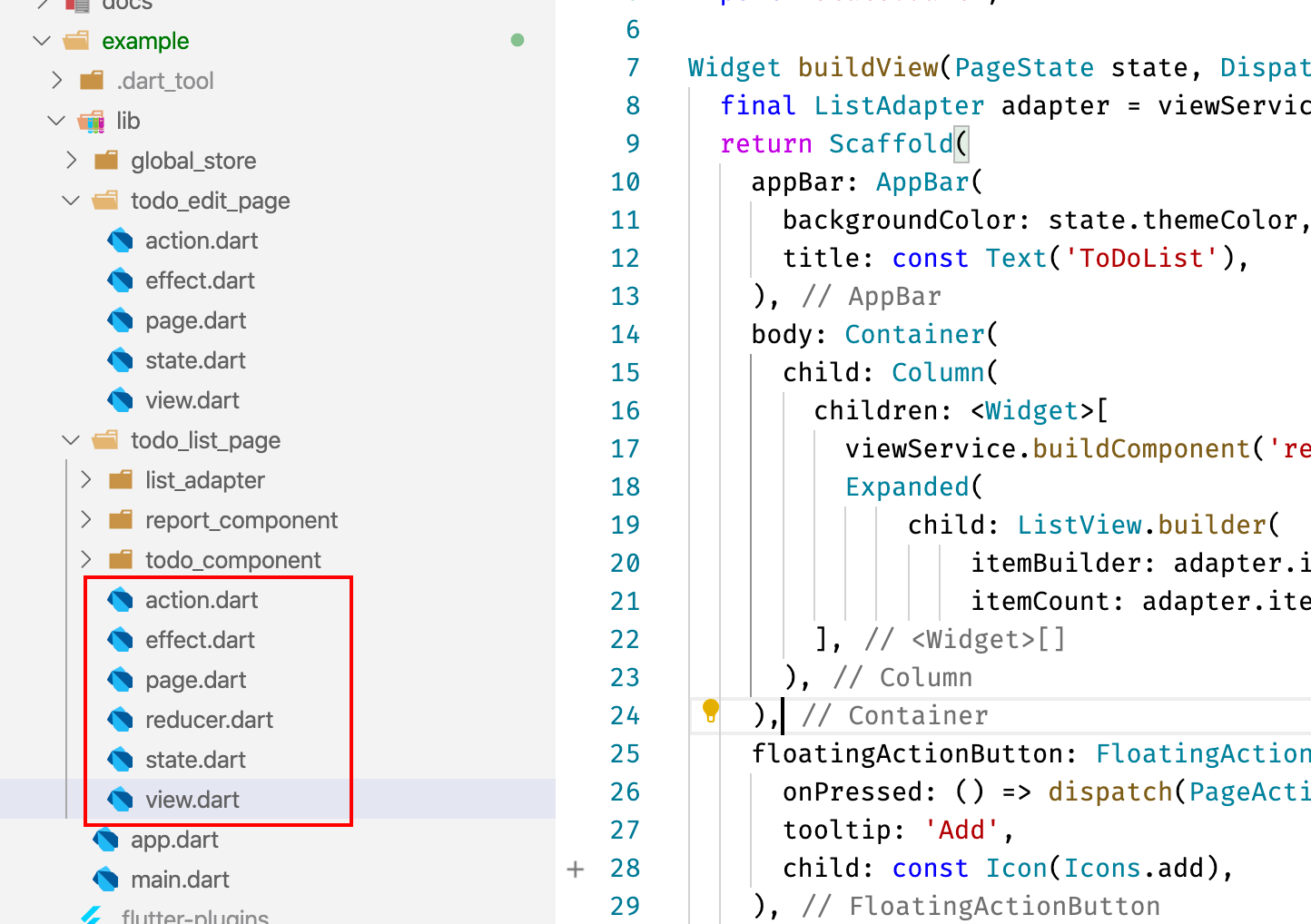
- fish-redux 代码
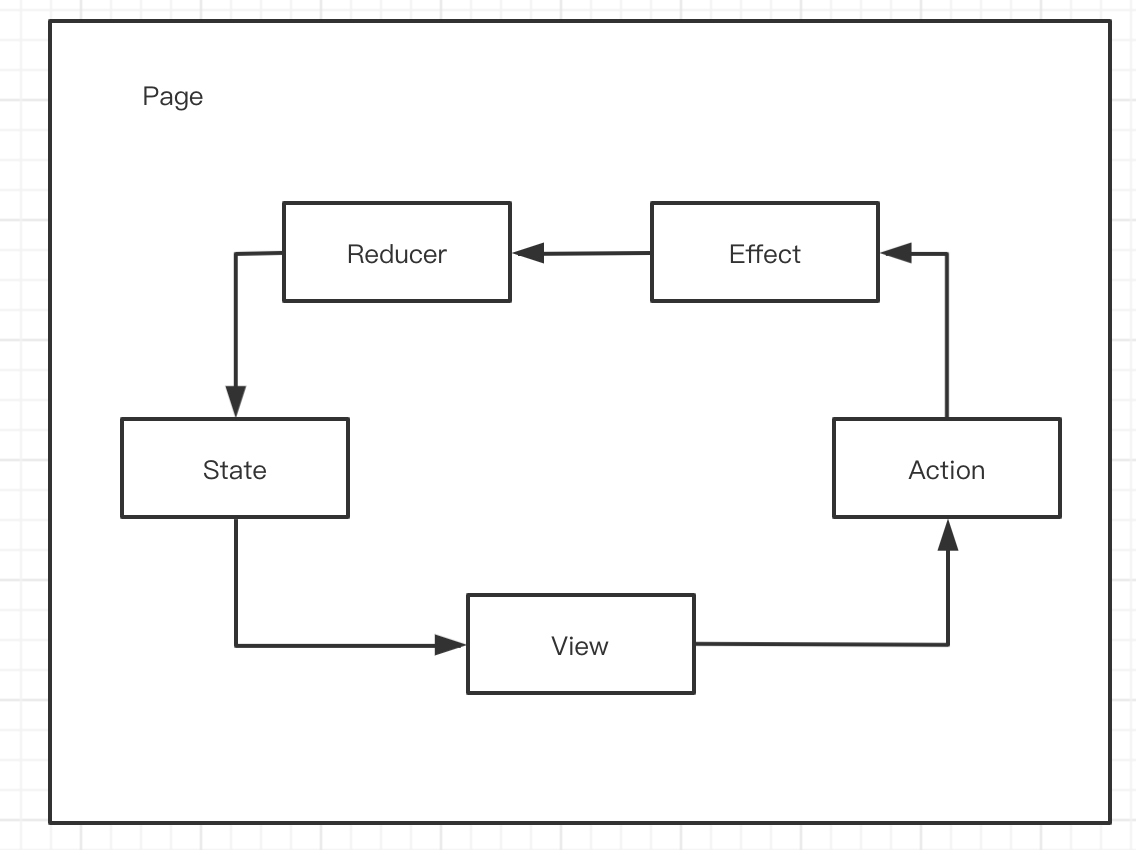
3.2 bloc
- 架构
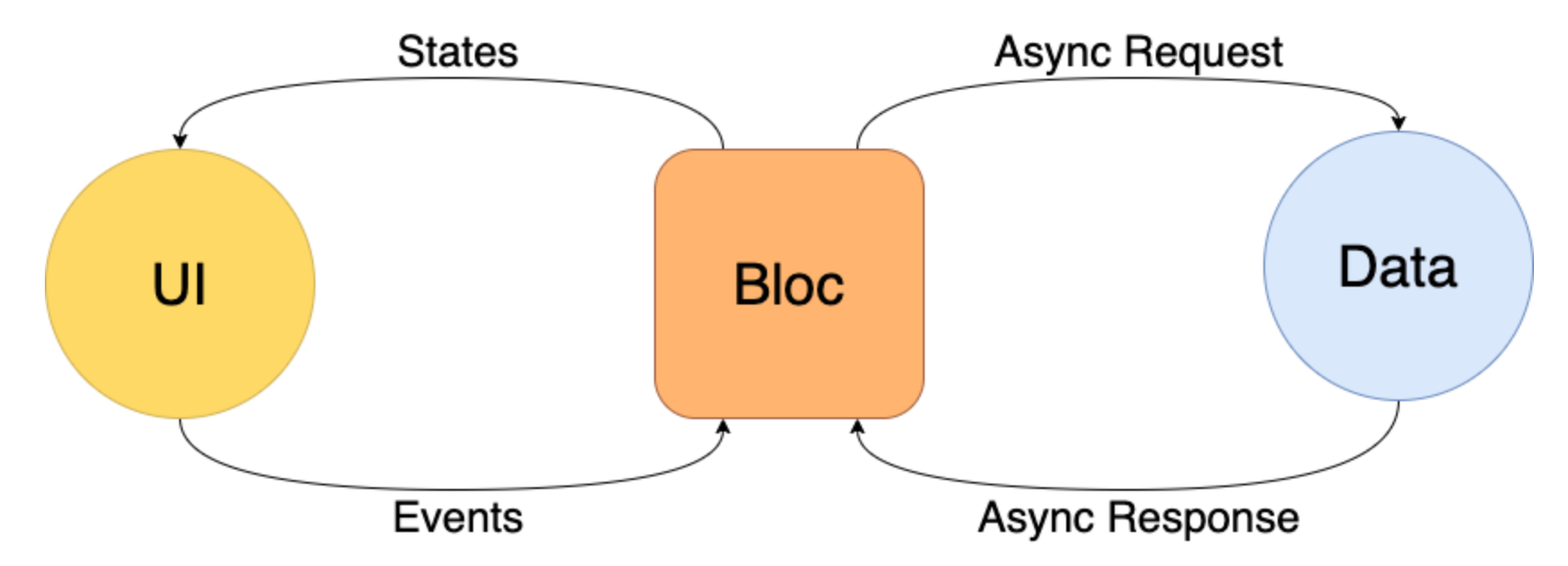
- 代码组织
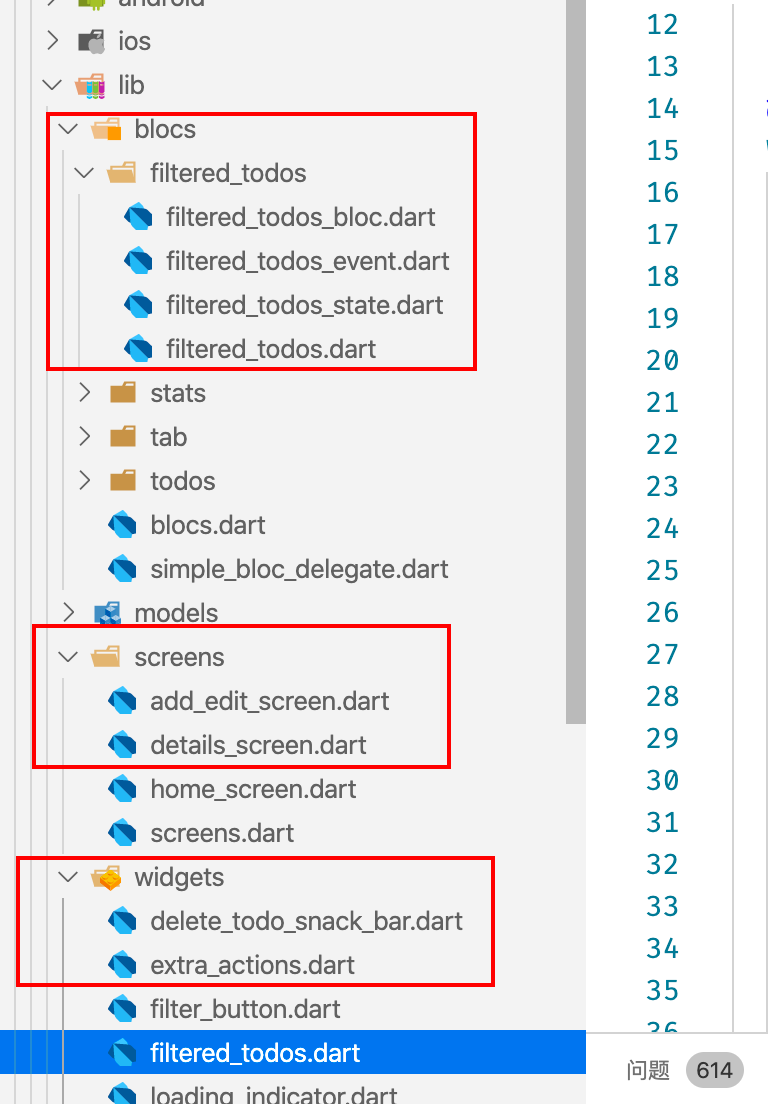
3.3 简单就是美
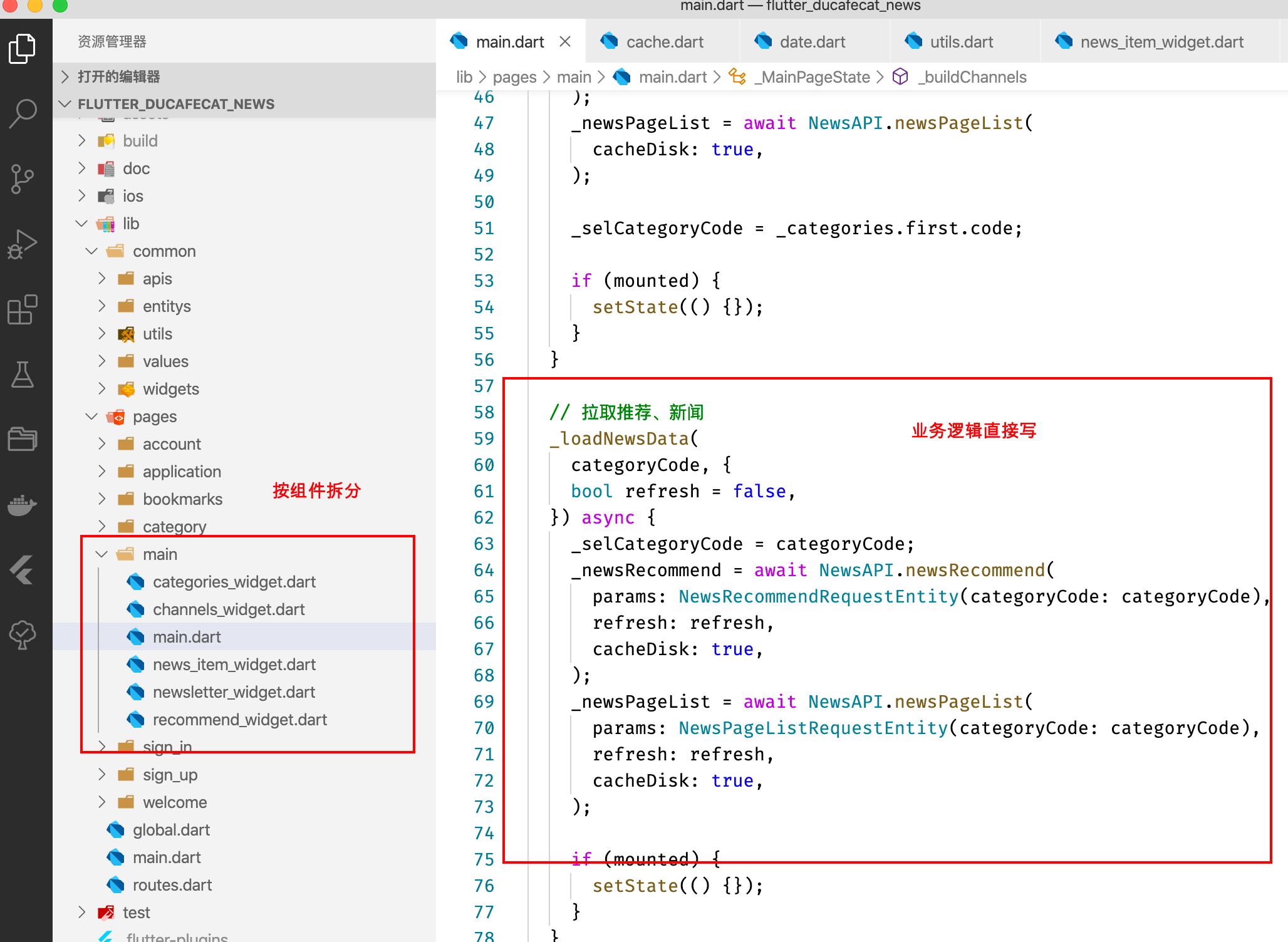
3.4 如何平衡
- 是否团队开发
- 是否简单业务(20 页面)
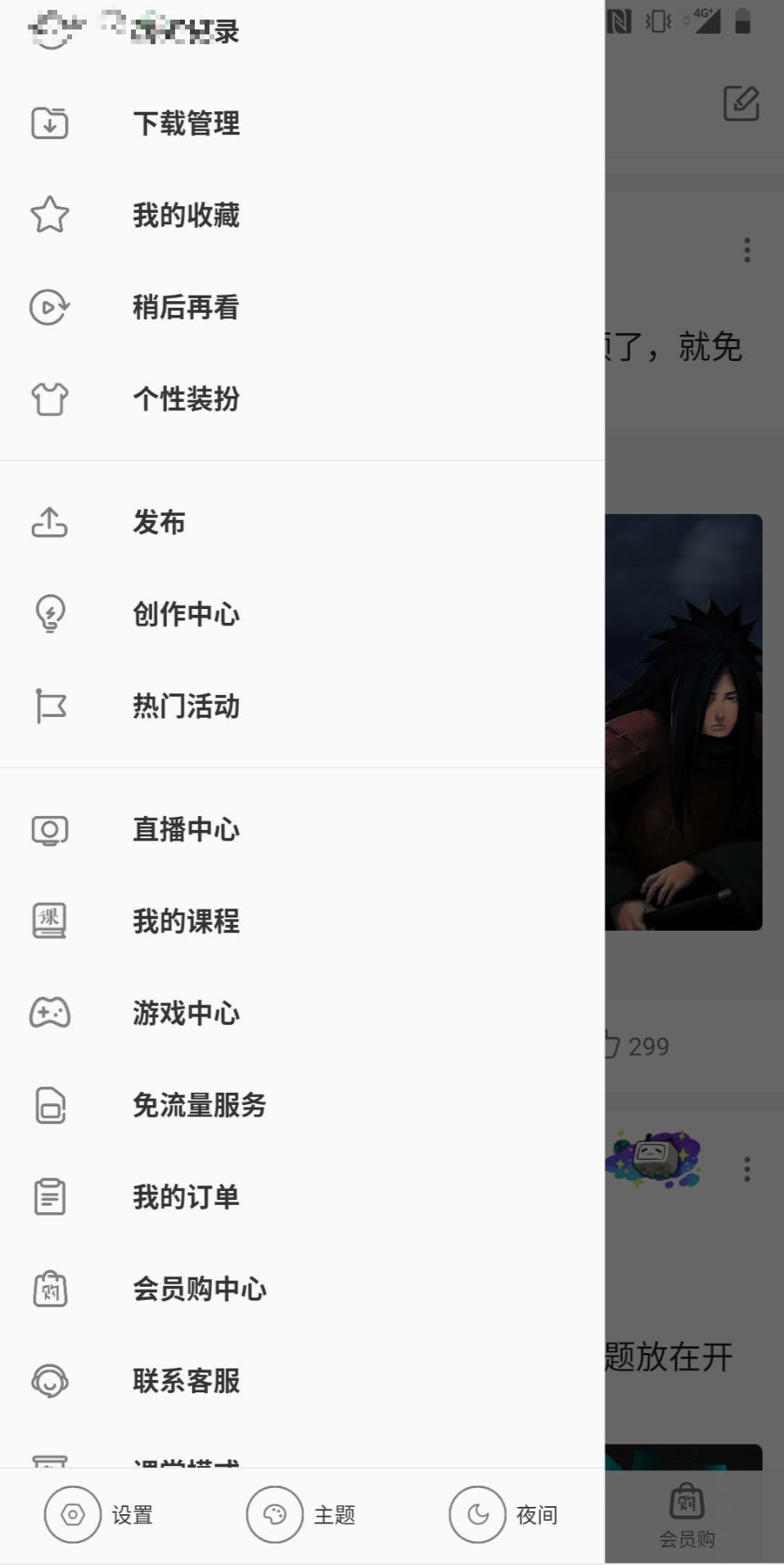
- 是否重交互(视频社交、聊天 A)
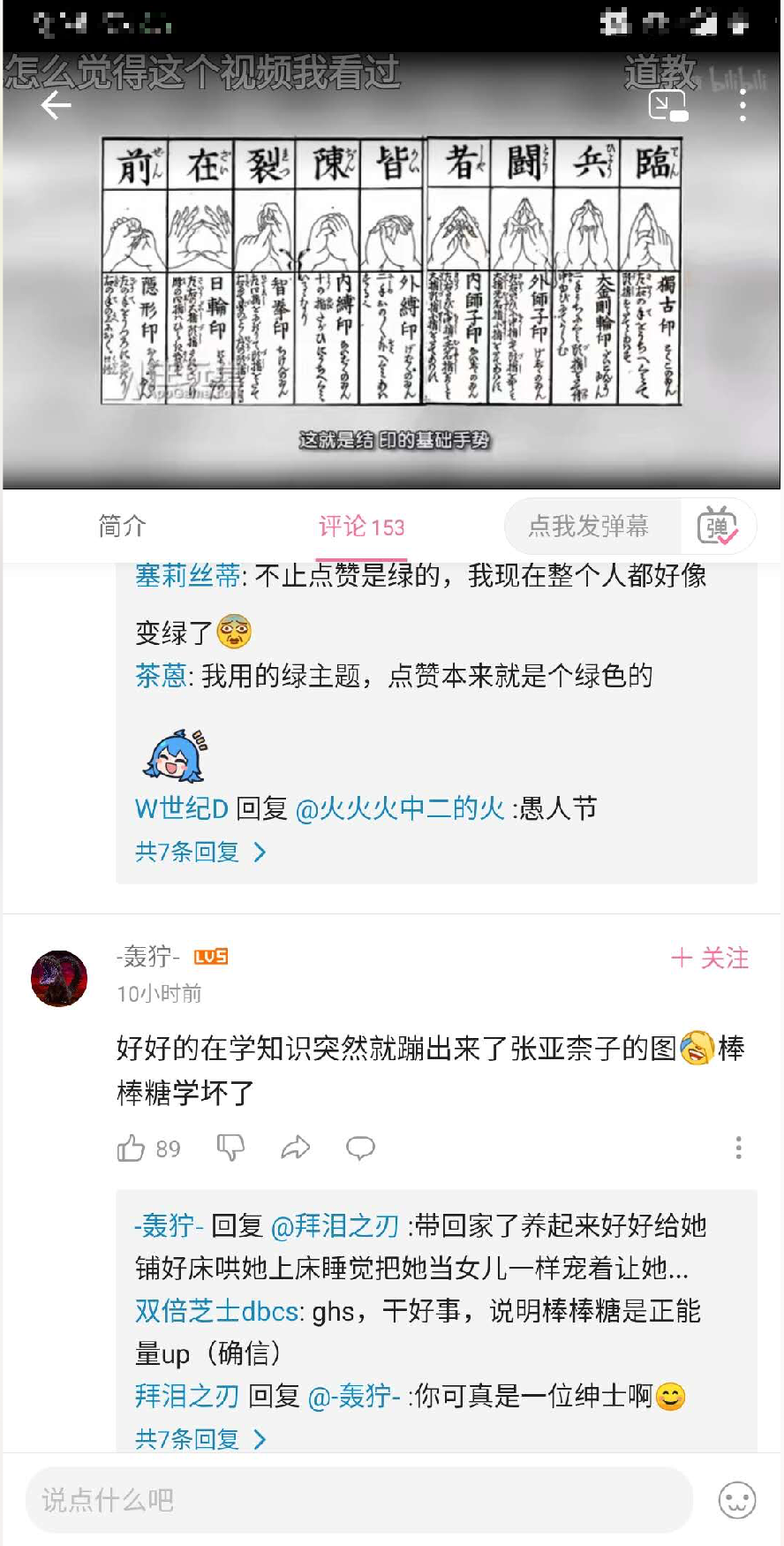
4 新闻首页实现
4.1 界面组成分析
- 分类导航、推荐新闻、频道导航
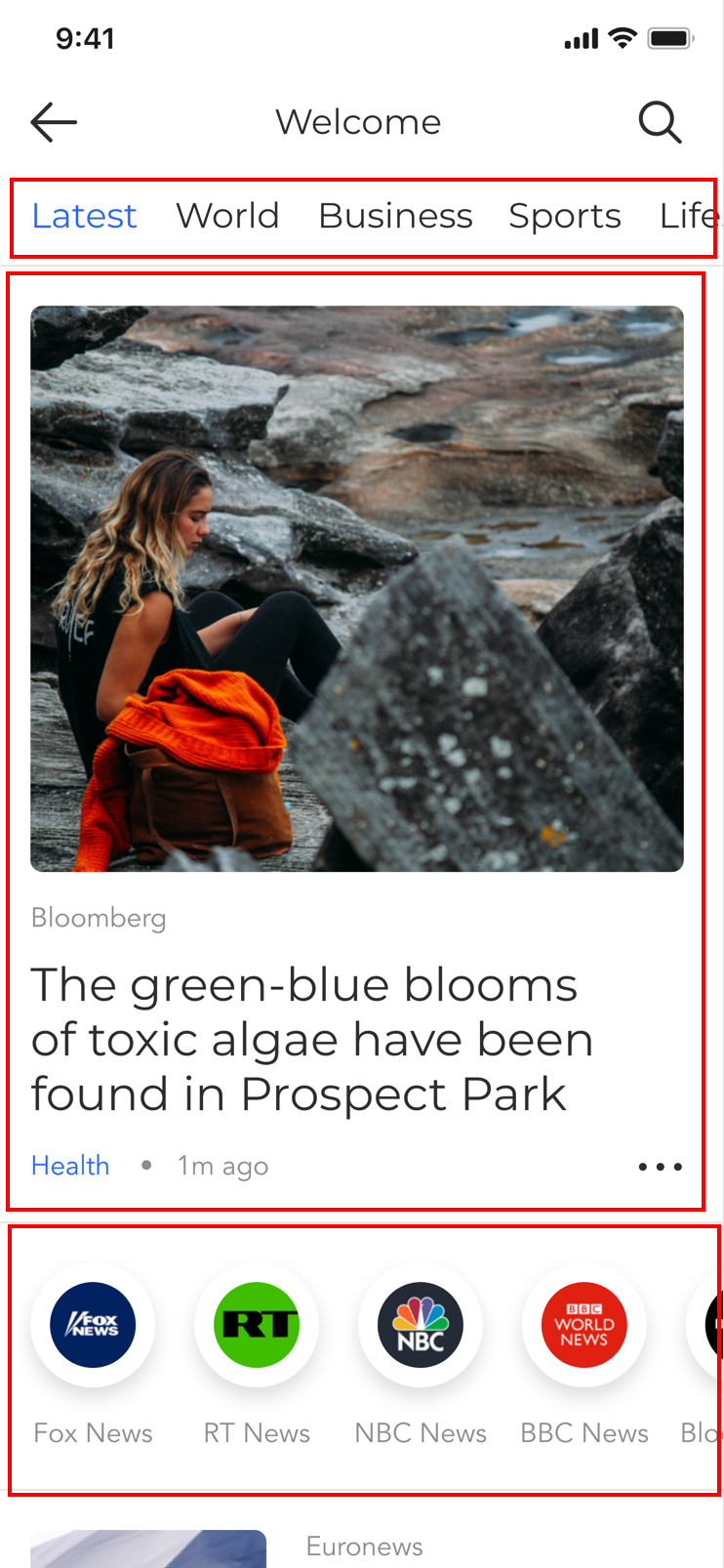
- 新闻列表、广告 ad、邮件订阅
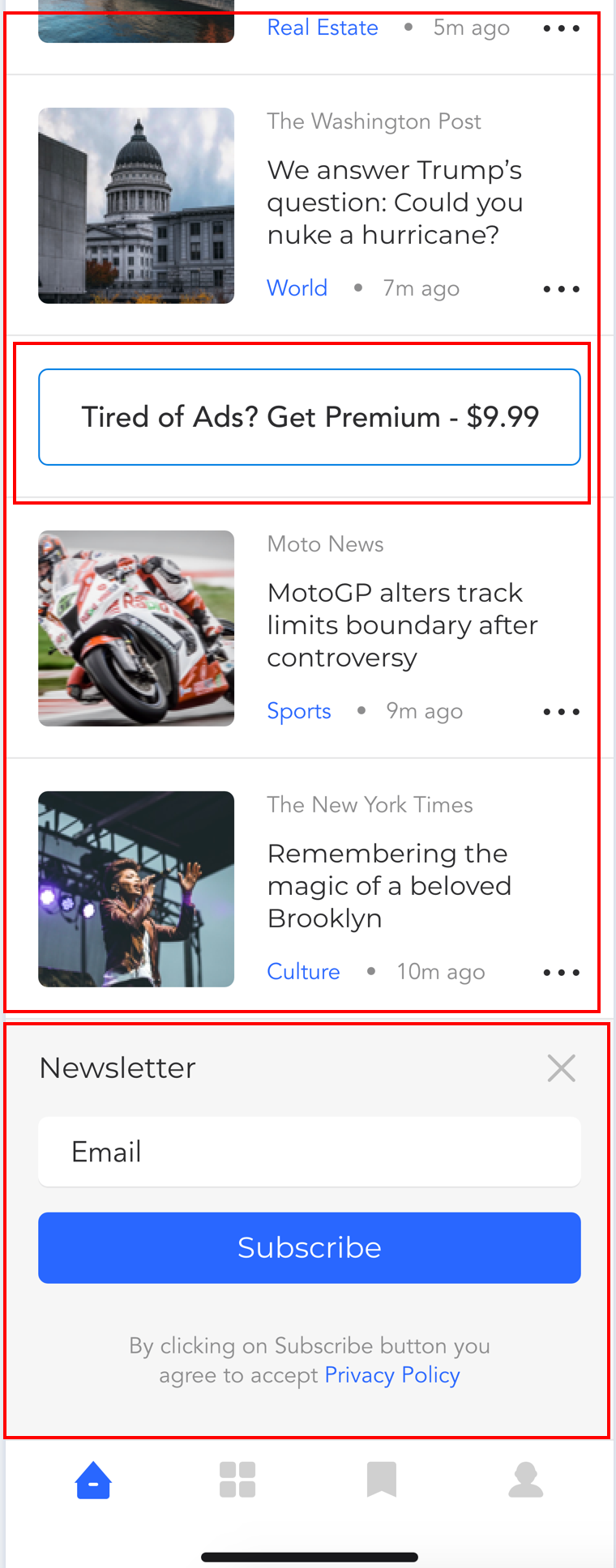
4.2 代码框架
1 | ... |
4.3 实现业务
- 创建 widget 单独文件
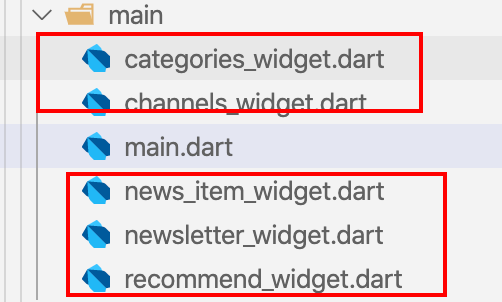
- 分类导航
lib/pages/main/categories_widget.dart
1 | Widget newsCategoriesWidget({ |
- 频道导航
lib/pages/main/channels_widget.dart
1 | Widget newsChannelsWidget({ |
- 新闻行 Item
lib/pages/main/news_item_widget.dart
1 | Widget newsItem(NewsItem item) { |
- 邮件订阅
lib/pages/main/newsletter_widget.dart
1 | Widget newsletterWidget() { |
- 推荐阅读
lib/pages/main/recommend_widget.dart
1 | Widget recommendWidget(NewsRecommendResponseEntity newsRecommend) { |
蓝湖设计稿
https://lanhuapp.com/url/lYuz1
密码: gSKl
蓝湖现在收费了,所以查看标记还请自己上传 xd 设计稿
商业设计稿文件不好直接分享, 可以加微信联系 ducafecat
YAPI 接口管理
参考
VSCode 插件
视频
© 猫哥